|
|
|
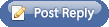 |

January 14th, 2007, 02:51 AM
|
 |
Colonel
|
|
Join Date: May 2005
Location: Kansas, USA
Posts: 1,538
Thanks: 289
Thanked 194 Times in 94 Posts
|
|
Simple Terrain Calculator...
Attached is a simple terrain calculator that I wrote to support my current side project. Nothing fancy, just enter a province terrain value and it lists the terrain flags. Might be handy for someone other than myself, and it is much faster than doing it manually.
You will need Yabasic which can be found here:
http://www.yabasic.de/
Yabasic runs under Unix and Windows; it is small (around 200KB) and free.
Last edited by Ballbarian; August 18th, 2008 at 09:26 PM..
|

January 14th, 2007, 07:20 AM
|
 |
Second Lieutenant
|
|
Join Date: Oct 2006
Location: Toulouse, FRANCE
Posts: 436
Thanks: 150
Thanked 21 Times in 13 Posts
|
|
Re: Simple Terrain Calculator...
For those that prefer Perl, here's my similar function (from my perl dom library project).
The function return an array with the list of all types.
Quote:
# extract list of terrain types from the numeric code
sub get_terrain_types_from_code($); # function prototype, prevent warning when the function try to call itself recursively in strict mode
sub get_terrain_types_from_code($)
{
my $terrain_code = shift;
if ($terrain_code !~ /^[0-9]+$/)
{
error("[get_terrain_types_from_code] terrain code must be numeric value ('$terrain_code')");
}
my @terrain_types = ();
# terrain code is the sum of the successives individual terrain type code values
if ($terrain_code >= 4194304) # "edgemount" 4194304
{
# code >= 4194304 means that province type include "edgemount"
push (@terrain_types, "edgemount");
# now get the other types (if any) by recursive call
push (@terrain_types, get_terrain_types_from_code($terrain_code - 4194304));
}
elsif ($terrain_code >= 2097152) # "priestsite" 2097152
{
# code >= 2097152 means that province type include "priestsite"
push (@terrain_types, "priestsite");
# now get the other types (if any) by recursive call
push (@terrain_types, get_terrain_types_from_code($terrain_code - 2097152));
}
elsif ($terrain_code >= 1048576) # "bloodsite" 1048576
{
# now you should know how it works ... ^_^
push (@terrain_types, "bloodsite");
# now get the other types (if any) by recursive call
push (@terrain_types, get_terrain_types_from_code($terrain_code - 1048576));
}
elsif ($terrain_code >= 524288) # "naturesite" 524288
{
# add the type to the list
push (@terrain_types, "naturesite");
# now get the other types (if any) by recursive call
push (@terrain_types, get_terrain_types_from_code($terrain_code - 524288));
}
elsif ($terrain_code >= 262144) # "deathsite" 262144
{
# add the type to the list
push (@terrain_types, "deathsite");
# now get the other types (if any) by recursive call
push (@terrain_types, get_terrain_types_from_code($terrain_code - 262144));
}
elsif ($terrain_code >= 131072) # "astralsite" 131072
{
# add the type to the list
push (@terrain_types, "astralsite");
# now get the other types (if any) by recursive call
push (@terrain_types, get_terrain_types_from_code($terrain_code - 131072));
}
elsif ($terrain_code >= 65536) # "earthsite" 65536
{
# add the type to the list
push (@terrain_types, "earthsite");
# now get the other types (if any) by recursive call
push (@terrain_types, get_terrain_types_from_code($terrain_code - 65536));
}
elsif ($terrain_code >= 32768) # "watersite" 32768
{
# add the type to the list
push (@terrain_types, "watersite");
# now get the other types (if any) by recursive call
push (@terrain_types, get_terrain_types_from_code($terrain_code - 32768));
}
elsif ($terrain_code >= 16384) # "airsite" 16384
{
# add the type to the list
push (@terrain_types, "airsite");
# now get the other types (if any) by recursive call
push (@terrain_types, get_terrain_types_from_code($terrain_code - 16384));
}
elsif ($terrain_code >= 8192) # "firesite" 8192
{
# add the type to the list
push (@terrain_types, "firesite");
# now get the other types (if any) by recursive call
push (@terrain_types, get_terrain_types_from_code($terrain_code - 8192));
}
elsif ($terrain_code >= 4096) # "cave" 4096
{
# add the type to the list (at last : a true terrain type !)
push (@terrain_types, "cave");
# now get the other types (if any) by recursive call
push (@terrain_types, get_terrain_types_from_code($terrain_code - 4096));
}
elsif ($terrain_code >= 2048) # "deep" 2048
{
# add the type to the list
push (@terrain_types, "deep");
# now get the other types (if any) by recursive call
push (@terrain_types, get_terrain_types_from_code($terrain_code - 2048));
}
elsif ($terrain_code >= 1024) # "manysites" 1024
{
# add the type to the list
push (@terrain_types, "manysites");
# now get the other types (if any) by recursive call
push (@terrain_types, get_terrain_types_from_code($terrain_code - 1024));
}
elsif ($terrain_code >= 512) # "nostart" 512
{
# add the type to the list
push (@terrain_types, "nostart");
# now get the other types (if any) by recursive call
push (@terrain_types, get_terrain_types_from_code($terrain_code - 512));
}
elsif ($terrain_code >= 256) # "farm" 256
{
# add the type to the list
push (@terrain_types, "farm");
# now get the other types (if any) by recursive call
push (@terrain_types, get_terrain_types_from_code($terrain_code - 256));
}
elsif ($terrain_code >= 128) # "forest" 128
{
# add the type to the list
push (@terrain_types, "forest");
# now get the other types (if any) by recursive call
push (@terrain_types, get_terrain_types_from_code($terrain_code - 128));
}
elsif ($terrain_code >= 64) # "waste" 64
{
# add the type to the list
push (@terrain_types, "waste");
# now get the other types (if any) by recursive call
push (@terrain_types, get_terrain_types_from_code($terrain_code - 64));
}
elsif ($terrain_code >= 32) # "swamp" 32
{
# add the type to the list
push (@terrain_types, "swamp");
# now get the other types (if any) by recursive call
push (@terrain_types, get_terrain_types_from_code($terrain_code - 32));
}
elsif ($terrain_code >= 16) # "mountain" 16
{
# add the type to the list
push (@terrain_types, "mountain");
# now get the other types (if any) by recursive call
push (@terrain_types, get_terrain_types_from_code($terrain_code - 16));
}
elsif ($terrain_code >= 8) # "somewater" 8
{
# add the type to the list
push (@terrain_types, "somewater");
# now get the other types (if any) by recursive call
push (@terrain_types, get_terrain_types_from_code($terrain_code - 8));
}
elsif ($terrain_code >= 4) # "sea" 4
{
# add the type to the list
push (@terrain_types, "sea");
# now get the other types (if any) by recursive call
push (@terrain_types, get_terrain_types_from_code($terrain_code - 4));
}
elsif ($terrain_code >= 2) # "large" 2
{
# add the type to the list
push (@terrain_types, "large");
# now get the other types (if any) by recursive call
push (@terrain_types, get_terrain_types_from_code($terrain_code - 2));
}
elsif ($terrain_code >= 1) # "small" 1
{
# add the type to the list
push (@terrain_types, "small");
# no other type, we're done
}
return @terrain_types;
}
|
|

January 14th, 2007, 03:31 PM
|
 |
Shrapnel Fanatic
|
|
Join Date: Oct 2003
Location: Vacaville, CA, USA
Posts: 13,736
Thanks: 341
Thanked 479 Times in 326 Posts
|
|
Re: Simple Terrain Calculator...
Quote:
Ballbarian said:
Attached is a simple terrain calculator that I wrote to support my current side project. Nothing fancy, just enter a province terrain value and it lists the terrain flags. Might be handy for someone other than myself, and it is much faster than doing it manually.
You will need Yabasic which can be found here:
http://www.yabasic.de/
Yabasic runs under Unix and Windows; it is small (around 200KB) and free.
|
Thank you Thank you.
Ive always hated bit-math
__________________
-- DISCLAIMER:
This game is NOT suitable for students, interns, apprentices, or anyone else who is expected to pass tests on a regular basis. Do not think about strategies while operating heavy machinery. Before beginning this game make arrangements for someone to check on you daily. If you find that your game has continued for more than 36 hours straight then you should consult a physician immediately (Do NOT show him the game!)
|
Posting Rules
|
You may not post new threads
You may not post replies
You may not post attachments
You may not edit your posts
HTML code is On
|
|
|
|
|